We believe that developers should focus on business code instead of wasting time on technical concerns.
Hero
Ogham is designed to get you up and running as quickly as possible, with minimal upfront configuration. Our aim is to provide a library that keeps developers focused on business code. Therefore, Ogham saves you painful steps. Developers don’t need to be experts in RFCs or low level protocols anymore. Even testing of message sending are simplified with Ogham.
Email, sms or whatever, Ogham provides everything to send any kind of messages. As Ogham is natively extensible, any kind of message that is not yet supported can be added easily.
Ogham is designed to work with real world applications. It integrates natively with most popular frameworks. In real world applications, sending email, sms or whatever often need to use an external service instead of the protocols. Ogham is designed to support several implementations for sending any kind of message. The aim is to code once and still be able to choose the implementation/protocol to use at runtime.
Usage
Send a simple email
Ogham provides a single entry point (MessagingService
) to send any message. To send an email, just prepare the email content using the Email
class. The fluent API lets you chain API calls and also helps to guide developers.
In standalone mode (without framework integration), MessagingService
has to be instantiated. In this sample, the predefined behavior is used. The properties are used to configure the SMTP protocol/implementation.
package fr.sii.ogham.sample.standard.email;
import java.util.Properties;
import fr.sii.ogham.core.builder.MessagingBuilder;
import fr.sii.ogham.core.exception.MessagingException;
import fr.sii.ogham.core.service.MessagingService;
import fr.sii.ogham.email.message.Email;
public class BasicSample {
public static void main(String[] args) throws MessagingException {
// configure properties (could be stored in a properties file or defined
// in System properties)
Properties properties = new Properties();
properties.put("mail.smtp.host", "<your server host>");
properties.put("mail.smtp.port", "<your server port>");
properties.put("ogham.email.from.default-value", "<email address to display for the sender user>");
// Instantiate the messaging service using default behavior and
// provided properties
MessagingService service = MessagingBuilder.standard() (1)
.environment()
.properties(properties) (2)
.and()
.build(); (3)
// send the email using fluent API
service.send(new Email() (4)
.subject("BasicSample")
.body().string("email content")
.to("ogham-test@yopmail.com"));
}
}
1 | Use the standard builder (predefined behavior) |
2 | Register the custom properties |
3 | Create a MessagingService instance |
4 | Send an email with a subject and a simple body. The sender address is automatically set using ogham.email.from.default-value property |
Send a simple SMS
As mentioned above, Ogham provides a single entrypoint. Sending SMS is exactly the same way as for email.
Prepare the SMS content and recipients using the Sms
class.
The standard protocol to send SMS is SMPP. Therefore to reach the SMPP server, properties are adapted to provide SMPP server configuration.
package fr.sii.ogham.sample.standard.sms;
import java.util.Properties;
import fr.sii.ogham.core.builder.MessagingBuilder;
import fr.sii.ogham.core.exception.MessagingException;
import fr.sii.ogham.core.service.MessagingService;
import fr.sii.ogham.sms.message.Sms;
public class BasicSample {
public static void main(String[] args) throws MessagingException {
// configure properties (could be stored in a properties file or defined
// in System properties)
Properties properties = new Properties();
properties.setProperty("ogham.sms.smpp.host", "<your server host>"); (1)
properties.setProperty("ogham.sms.smpp.port", "<your server port>"); (2)
properties.setProperty("ogham.sms.smpp.system-id", "<your server system ID>"); (3)
properties.setProperty("ogham.sms.smpp.password", "<your server password>"); (4)
properties.setProperty("ogham.sms.from.default-value", "<phone number to display for the sender>"); (5)
// Instantiate the messaging service using default behavior and
// provided properties
MessagingService service = MessagingBuilder.standard() (6)
.environment()
.properties(properties) (7)
.and()
.build(); (8)
// send the sms using fluent API
service.send(new Sms() (9)
.message().string("sms content")
.to("+33752962193"));
}
}
1 | Configure the SMPP server host |
2 | Configure the SMPP server port |
3 | The SMPP system ID |
4 | The SMPP password |
5 | The phone number of the sender |
6 | Use the standard builder (predefined behavior) |
7 | Register the custom properties |
8 | Create a MessagingService instance |
9 | Send a SMS with a simple message. The sender phone number is automatically set using ogham.sms.from.default-value property |
Send using a template
In real world applications, messages content need to be constructed with variable parts. That’s why Ogham provides integration with several templating engines.
The only difference here is the use of TemplateContent
instead of a string. This indicates to Ogham that the content is a template to evaluate.
The template is located in the classpath (use of classpath:
). The template contains variables (name
and value
). To evaluate the template, a model is needed to provide values for name
and value
. In the example, the class SimpleBean
is defined with the properties and used as model.
Templates can be used for SMS or whatever in the same way.
In the sample, Thymeleaf template engine is used but it could be any template engine.
package fr.sii.ogham.sample.standard.email;
import java.util.Properties;
import fr.sii.ogham.core.builder.MessagingBuilder;
import fr.sii.ogham.core.exception.MessagingException;
import fr.sii.ogham.core.service.MessagingService;
import fr.sii.ogham.email.message.Email;
public class HtmlTemplateSample {
public static void main(String[] args) throws MessagingException {
// configure properties (could be stored in a properties file or defined
// in System properties)
Properties properties = new Properties();
properties.setProperty("mail.smtp.host", "<your server host>");
properties.setProperty("mail.smtp.port", "<your server port>");
properties.setProperty("ogham.email.from.default-value", "<email address to display for the sender user>");
// Instantiate the messaging service using default behavior and
// provided properties
MessagingService service = MessagingBuilder.standard() (1)
.environment()
.properties(properties) (2)
.and()
.build(); (3)
// send the email using fluent API
service.send(new Email() (4)
.subject("HtmlTemplateSample")
.body().template("classpath:/template/thymeleaf/simple.html", (5)
new SimpleBean("foo", 42)) (6)
.to("ogham-test@yopmail.com"));
}
public static class SimpleBean {
private String name;
private int value;
public SimpleBean(String name, int value) {
super();
this.name = name;
this.value = value;
}
public String getName() {
return name;
}
public int getValue() {
return value;
}
}
}
1 | Use the standard builder (predefined behavior) |
2 | Register the custom properties |
3 | Create a MessagingService instance |
4 | Send an email with a subject and a simple body that comes from the evaluated template. The sender address is automatically set using ogham.email.from.default-value property |
5 | Indicate the path to the HTML template file (in the classpath) |
6 | Use any bean object for replacing variables in template |
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org"> (1)
<head>
<meta charset="utf-8" />
</head>
<body>
<h1 class="title" th:text="${name}"></h1> (2)
<p class="text" th:text="${value}"></p> (3)
</body>
</html>
1 | Include the ThymeLeaf namespace |
2 | Use the name attribute value in the template |
3 | Use the value attribute value in the template |
Get it now
Standalone usage
ogham-all
dependency provides all Ogham features. All implementations/protocols are included for all kind of messages. All supported templated engines are also included.
The aim is to start quickly and avoid wasting time on choosing dependencies.
Later, developers can adapt included features by selecting Ogham dependencies (no need to exclude some dependencies).
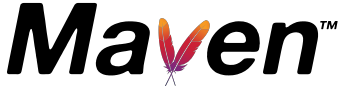
ogham-all
dependency to your pom.xml
<dependency>
<groupId>fr.sii.ogham</groupId>
<artifactId>ogham-all</artifactId>
<version>3.0.0</version>
</dependency>
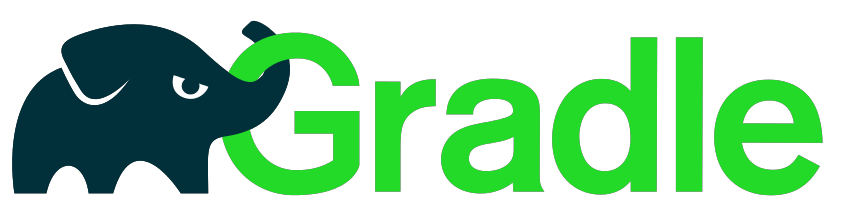
ogham-all
dependency to your build.gradle
dependencies {
implementation 'fr.sii.ogham:ogham-all:3.0.0'
}
Spring Boot starter
Ogham natively provides Spring Boot integration. ogham-spring-boot-starter-all
is like ogham-all
but uses Spring Boot starters instead for implementations/template engines.
Developers can also select features by selecting Ogham dependencies for Spring Boot.
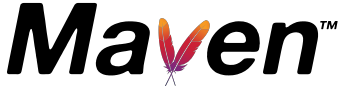
ogham-spring-boot-starter-all
dependency to your pom.xml
<dependency>
<groupId>fr.sii.ogham</groupId>
<artifactId>ogham-spring-boot-starter-all</artifactId>
<version>3.0.0</version>
</dependency>
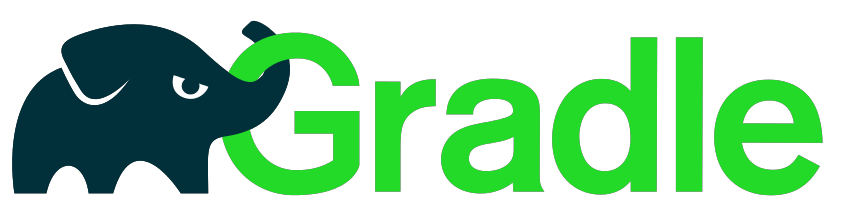
ogham-spring-boot-starter-all
dependency to your build.gradle
dependencies {
implementation 'fr.sii.ogham:ogham-spring-boot-starter-all:3.0.0'
}