Send Email
Features
Send SMS
-
No need to know SMPP protocol
-
Send SMS through SMPP (using Cloudhopper, through SmsGlobal for example) or HTTP (through OVH)
Designed for templating
-
Easy file loading
-
ThymeLeaf integration
-
FreeMarker integration
Framework support
-
-
Spring Boot starter
-
Compatible with other Spring Boot modules
-
Java EE
Testing tools
Automatic configuration
-
Protocol selection based on classpath and configuration
-
Template engine selection based on classpath and template content
Existing libraries
Several libraries for sending email already exist:
These libraries help you send an email but if you want to use a templated content, you will have to manually integrate a template engine.
These libraries also provide only implementations based on Java Mail API. But in some environments, you might NOT want to send the email directly but to use a web service to do it for you (SendGrid for example). Furthermore, those libraries are bound by design to frameworks or libraries that you might not want to use in your own context.
So, now you would want to find a sending library with a high level of abstraction to avoid binding issues with any template engine, design framework or sender service… Is email the only possible message type ? No, so why not sending SMS, Tweet or anything the same way ?
The Ogham module
This module is designed for handling any kind of message the same way. It also provides several implementations for the same message type. It selects the best implementation based on the classpath or properties for example. You can easily add your own implementation.
It also provides templating support and integrates natively several template engines. You can also add your own.
It is framework and library agnostic and provides bridges for common frameworks integration (Spring, JSF, …).
When using the module to send email based on an HTML template, the templating system let you design your HTML like a standard HTML page. It automatically transforms the associated resources (images, css files…) to be usable in an email context (automatic inline css, embed images…). You don’t need to write your HTML specifically for email.
Code once, adapt to environment
You write your business code once. Ogham handles environment switching just by providing different configuration:
Switch sender according to environment (tests/dev/prod)
Development
In development phase, you may want to send email through any SMTP server (GMail for example) and SMS through any SMPP server (SmsGlobal for example). Your templates can be embedded in classpath.
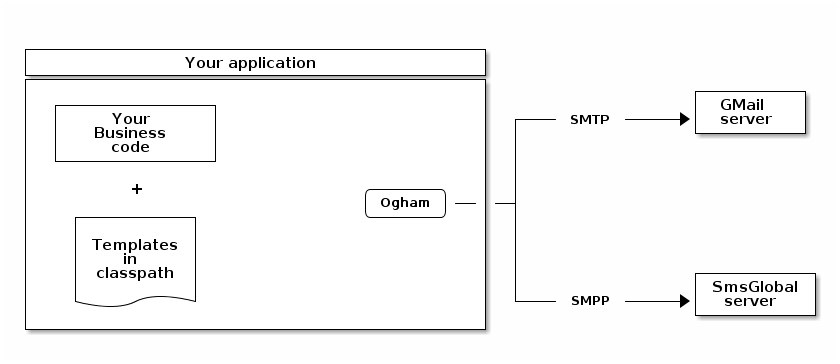
Tests
You can test your emails content through an embedded local SMTP. You can do the same with SMS through an embedded local SMPP server.
Ogham directly provides embedded servers for testing. Your templates can be embedded in test sources.
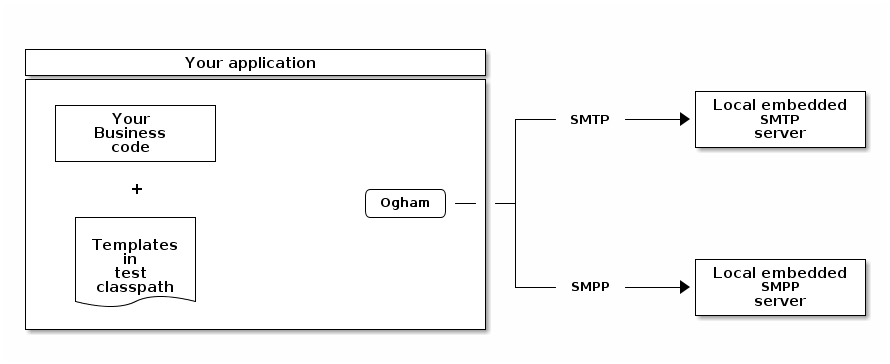
Production
In production, your enterprise may use external services for sending emails or SMS (like SendGrid HTTP API for emails and OVH HTTP API for SMS).
Externalizing templates let the possibility to update them without needing to deploy the application again.
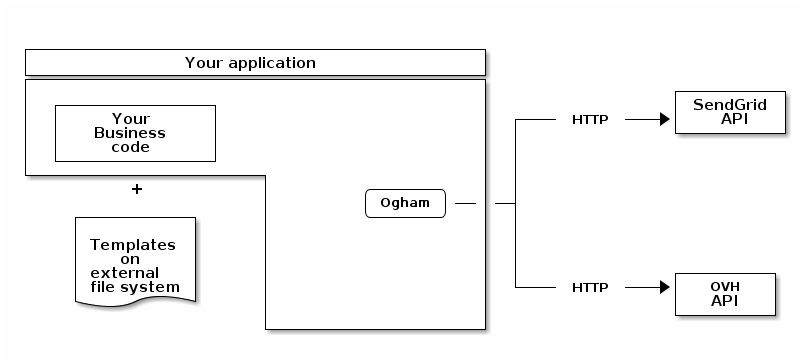
-
In all cases, the code never changes. Only Ogham configuration differs.
Send Email
package fr.sii.ogham.sample.standard.email;
import java.util.Properties;
import fr.sii.ogham.core.builder.MessagingBuilder;
import fr.sii.ogham.core.exception.MessagingException;
import fr.sii.ogham.core.service.MessagingService;
import fr.sii.ogham.email.message.Email;
public class BasicSample {
public static void main(String[] args) throws MessagingException {
// configure properties (could be stored in a properties file or defined
// in System properties)
Properties properties = new Properties();
properties.put("mail.smtp.host", "<your server host>");
properties.put("mail.smtp.port", "<your server port>");
properties.put("ogham.email.from", "<email address to display for the sender user>");
// Instantiate the messaging service using default behavior and
// provided properties
MessagingService service = MessagingBuilder.standard() (1)
.environment()
.properties(properties) (2)
.and()
.build(); (3)
// send the email using fluent API
service.send(new Email() (4)
.subject("subject")
.content("email content")
.to("ogham-test@yopmail.com"));
}
}
1 | Use the standard builder (predefined behavior) |
2 | Register the custom properties |
3 | Create a MessagingService instance |
4 | Send an email with a subject and a simple body. The sender address is automatically set using ogham.email.from property |
Send SMS
package fr.sii.ogham.sample.standard.sms;
import java.util.Properties;
import fr.sii.ogham.core.builder.MessagingBuilder;
import fr.sii.ogham.core.exception.MessagingException;
import fr.sii.ogham.core.service.MessagingService;
import fr.sii.ogham.sms.message.Sms;
public class BasicSample {
public static void main(String[] args) throws MessagingException {
// configure properties (could be stored in a properties file or defined
// in System properties)
Properties properties = new Properties();
properties.setProperty("ogham.sms.smpp.host", "<your server host>"); (1)
properties.setProperty("ogham.sms.smpp.port", "<your server port>"); (2)
properties.setProperty("ogham.sms.smpp.system-id", "<your server system ID>"); (3)
properties.setProperty("ogham.sms.smpp.password", "<your server password>"); (4)
properties.setProperty("ogham.sms.from", "<phone number to display for the sender>"); (5)
// Instantiate the messaging service using default behavior and
// provided properties
MessagingService service = MessagingBuilder.standard() (6)
.environment()
.properties(properties) (7)
.and()
.build(); (8)
// send the sms using fluent API
service.send(new Sms() (9)
.content("sms content")
.to("+33752962193"));
}
}
1 | Configure the SMPP server host |
2 | Configure the SMPP server port |
3 | The SMPP system ID |
4 | The SMPP password |
5 | The phone number of the sender |
6 | Use the standard builder (predefined behavior) |
7 | Register the custom properties |
8 | Create a MessagingService instance |
9 | Send a SMS with a simple message. The sender phone number is automatically set using ogham.sms.from property |
Standalone usage
To use Ogham without any framework:
Include Ogham standalone dependency
<dependency>
<groupId>fr.sii.ogham</groupId>
<artifactId>ogham-all</artifactId>
<version>2.0.0</version>
</dependency>
-