Spring Boot 2.1.x - Date : November 2018
What is Spring Boot ?
- Built on top of a lot of Spring Projects (https://spring.io)
- Opinionated configuration
- Wide ecosystem
- Start a project faster with no configuration
Features
- Bootstrap class
SpringApplication
- Default logger (@see spring-jcl)
- FailureAnalyzers : friendly failure report
- Application Events on Listeners
- Choose the right
ApplicationContext
- Accessing application arguments
- Control application exit code
Quickstart
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
</dependencies>
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
Best practices
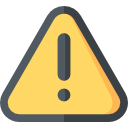
Code structure
com
+ example
| + project
| | - MyApp.java
| | |
| | + player
| | | - Player.java
| | | - PlayerService.java
Configuration
Properties files in folder src/main/resources/ are loaded automatically
- YAML :
**/application*.yml
or**/application*.yaml
- Properties :
**/application*.properties
Auto-configuration
- Enabled by
@SpringBootApplication
or@EnableAutoConfiguration
- Spring Boot scans all libs on the classpath and auto-configures them (if you didn't manually)
Display Spring Boot Autoconfigure report
java -jar myapp.jar --debug
Disabling an auto-configuration class with Java conf
@EnableAutoConfiguration(exclude={DataSourceAutoConfiguration.class})
Disabling an auto-configuration class with properties
spring.autoconfigure.exclude= \
org.springframework.boot.autoconfigure.XXXX